class Solution {
public:
vector<vector<int>> spiralMatrixIII(int rows, int cols, int rStart, int cStart) {
int m = rows;
int n = cols;
vector<vector<int>> ans;
vector<vector<int>> vis(m+n, vector<int>(m+n, 0));
int x = rStart;
int y = cStart;
int k = 1;
while(ans.size()<m*n){
// top row
for(int j = y; j<y+k; j++){
if(x>=0 && j>=0 && x<m && j<n && !vis[x][j]){
vis[x][j] = 1;
ans.push_back({x, j});
}
}
y+=k;
// right col
for(int i = x; i<x+k; i++){
if(i>=0 && y>=0 && i<m && y<n && !vis[i][y]){
vis[i][y] = 1;
ans.push_back({i, y});
}
}
x+=k;
k++;
//bottm row
for(int j = y; j>=y-k; j--){
if(x>=0 && j>=0 && x<m && j<n && !vis[x][j]){
vis[x][j] = 1;
ans.push_back({x, j});
}
}
y-=k;
// left col
for(int i = x; i>=x-k; i--){
if(i>=0 && y>=0 && i<m && y<n && !vis[i][y]){
vis[i][y] = 1;
ans.push_back({i, y});
}
}
x-=k;
k++;
}
return ans;
}
};
LeetCode, GeeksForGeeks Problem of the day solution

Channel link :
https://t.me/leetcode_gfg_potd
Similar Channels


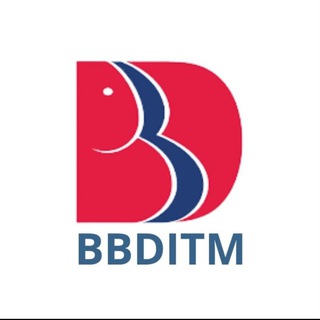
Daily Challenges on LeetCode and GeeksForGeeks: Unlocking the Code
In the ever-evolving field of software development, continuous learning and practice are crucial for mastering programming languages and concepts. Two prominent platforms that cater to these needs are LeetCode and GeeksForGeeks, both of which offer a variety of daily challenges that not only test your problem-solving skills but also provide rewards for consistent participation. These platforms have gained immense popularity among coders, computer science students, and professionals, offering a wide range of problems that cover essential topics in algorithms and data structures. By engaging with these daily challenges, participants can not only solidify their understanding of core concepts but also prepare for technical interviews, competitions, and coding assessments. Furthermore, community-driven initiatives, such as the Telegram channel '@leetcode_gfg_potd', serve as a hub for sharing solutions, discussing strategies, and redeeming rewards, fostering an environment of collaborative learning and engagement among coding enthusiasts.
What types of challenges are available on LeetCode and GeeksForGeeks?
LeetCode and GeeksForGeeks provide a diverse array of challenges ranging from beginner to advanced levels. These challenges encompass various topics such as arrays, strings, trees, graphs, dynamic programming, and more. Each platform has its unique strengths; for instance, LeetCode is well-known for its extensive collection, particularly tailored for technical interviews, while GeeksForGeeks offers a wide range of problems along with comprehensive tutorials and discussion forums.
Moreover, both platforms often feature 'problem of the day' segments that encourage users to solve a new challenge each day. This initiative not only promotes daily practice but also helps users gradually build their skills. As participants tackle these problems, they can learn from the community through shared solutions and explanations, enhancing their problem-solving techniques.
How do daily challenges improve coding skills?
Participating in daily coding challenges fosters a habit of regular practice, which is essential for mastering programming concepts. By solving problems consistently, developers can identify their weaknesses and areas for improvement, allowing them to focus on specific topics that require more attention. This iterative practice helps solidify knowledge, making it easier to recall concepts during real-world application or under interview pressure.
Furthermore, these challenges often present varied approaches to problem-solving, encouraging participants to explore different algorithms and data structures. This exposure not only broadens their understanding but also sharpens critical thinking skills, enabling them to tackle complex problems effectively in their future endeavors.
What rewards can participants earn from these challenges?
Both LeetCode and GeeksForGeeks have implemented incentive systems that reward participants for their dedication and performance in daily challenges. On LeetCode, users can earn points, badges, and even premium memberships, which grant access to exclusive content and features. This gamification aspect motivates users to engage more frequently with the platform and strive for excellence in their coding skills.
Similarly, GeeksForGeeks may offer rewards through its community initiatives, including recognition in forums, potential job opportunities, and access to exclusive webinars or workshops. These benefits highlight the value of consistent participation, reinforcing the idea that commitment to learning can yield tangible results.
How can users effectively utilize the '@leetcode_gfg_potd' Telegram channel?
The '@leetcode_gfg_potd' Telegram channel serves as a valuable resource for participants in the daily challenges offered by LeetCode and GeeksForGeeks. Users can join this channel to receive regular updates on the problem of the day, allowing them to stay engaged and compete with a community of like-minded developers. This social aspect of problem-solving can enhance motivation and create a sense of camaraderie as users share their progress and challenges.
Additionally, the channel often facilitates discussions around strategies, solutions, and resources that can benefit all participants. By engaging with others, users can gain insights into different problem-solving techniques while also contributing their knowledge, making the learning experience more comprehensive and collaborative.
What is the best way to approach solving problems on these platforms?
To successfully tackle problems on LeetCode and GeeksForGeeks, users should first read the problem statement carefully to understand the requirements and constraints. It can be beneficial to break the problem down into smaller components and then devise a plan for solving each part. Many experienced coders recommend starting with a brute-force approach before optimizing their solution to improve efficiency.
After coding the solution, it's crucial to test it against various test cases, especially edge cases that might not be immediately apparent. Leveraging the community discussions and solutions posted on both platforms can also provide inspiration and alternative methods, further enhancing one's coding prowess.
LeetCode, GeeksForGeeks Problem of the day solution Telegram Channel
Are you someone who loves a good challenge and enjoys solving problems? Look no further than the LeetCode, GeeksForGeeks Problem of the day solution channel! This channel is dedicated to providing you with daily challenges from LeetCode and GeeksForGeeks, two of the most popular platforms for coding practice and problem-solving.
Whether you are a seasoned coder looking to hone your skills or a beginner eager to learn and improve, this channel is perfect for you. Each day, you will be presented with a new problem to solve, giving you the opportunity to test your knowledge and problem-solving abilities. And the best part? You can redeem rewards for completing these challenges, adding an extra layer of motivation and excitement to your coding journey.
By joining the LeetCode, GeeksForGeeks Problem of the day solution channel, you will have access to a supportive community of like-minded individuals who share your passion for coding and problem-solving. You can exchange ideas, ask for help, and celebrate your successes together, making the learning process even more enjoyable and rewarding.
Don't miss out on this fantastic opportunity to challenge yourself, improve your coding skills, and earn rewards along the way. Join the LeetCode, GeeksForGeeks Problem of the day solution channel today by clicking on the link below:
Channel link :
https://t.me/leetcode_gfg_potd