C , C++ PROGRAMMING LANGUAGES WITH DSA 💻
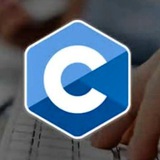
Disclaimer-
NO COPYRIGHT CONTENT IS UPLOADED HERE
THIS IS ONLY FOR EDUCATIONAL PURPOSE
#c_programming_language
#cpp #dsa #datastructuresalgorithms
#faang #c++
Similar Channels


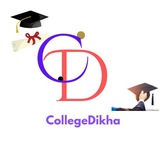
Understanding C and C++ Programming Languages with Data Structures and Algorithms
C and C++ are two of the most widely used programming languages in the world of software development. Developed in the 1970s, C was designed for system programming and embedded systems, while C++ emerged as an extension of C, introducing object-oriented programming features. Both languages are known for their performance efficiency, control over system resources, and their ability to manage complex data structures, which is fundamental in the field of data structures and algorithms (DSA). DSA is considered a core foundation of computer science, encompassing various techniques for organizing and processing data efficiently. This article will explore the significance of C and C++ in programming, their applications in DSA, and their impact on the tech industry, especially in high-demand environments like FAANG companies (Facebook, Apple, Amazon, Netflix, Google).
What is the difference between C and C++ programming languages?
C is a procedural programming language, which means it follows a step-by-step approach to writing code. It is built around functions, and its focus is on the sequence of actions that lead to a result. In contrast, C++ is a multi-paradigm programming language that supports both procedural and object-oriented programming. This means that in C++, developers can create objects that represent real-world entities and manipulate them through methods, leading to more modular and maintainable code.
Additionally, C++ introduces features like classes, inheritance, polymorphism, and encapsulation, enhancing code reusability and abstraction. While C is often used for system-level programming, C++ is preferred for applications that require a high level of complexity and abstraction, such as game development, GUI applications, and high-performance software.
How do data structures and algorithms relate to C and C++?
Data structures are specific ways to organize and store data in a computer so that it can be accessed and modified efficiently. Algorithms are step-by-step procedures or formulas for solving problems. C and C++ are particularly well-suited for implementing various data structures, such as arrays, linked lists, stacks, queues, trees, and graphs, due to their low-level memory management capabilities.
In C, developers have direct access to memory via pointers, allowing for efficient manipulation of data structures. C++ enhances this with Standard Template Library (STL), which provides a collection of ready-to-use data structures and algorithms. This library simplifies coding by offering well-tested components, making it easier for developers to implement complex data structures and algorithms without starting from scratch.
Why are C and C++ popular choices for teaching data structures and algorithms?
C and C++ are favored in academic settings for teaching data structures and algorithms because they provide a clear understanding of how data is managed in memory. Learning these languages helps students grasp fundamental concepts like pointers, dynamic memory allocation, and data representation, which are crucial for mastering DSA.
Moreover, both languages are widely used in industry, particularly in sectors requiring high-performance computing, such as gaming, embedded systems, and systems programming. By learning C and C++, students are better prepared for careers in software development and are equipped with the skills necessary to tackle real-world programming challenges.
What role do C and C++ play in modern software development?
C and C++ continue to play a significant role in modern software development due to their performance, efficiency, and versatility. They are extensively used in developing operating systems, game engines, high-frequency trading platforms, and applications requiring real-time processing due to their ability to execute close to the hardware layer.
Additionally, many modern languages and frameworks are built upon C and C++, making them foundational languages in the tech industry. Understanding these languages enables developers to comprehend underlying technologies, optimize code performance, and make informed decisions regarding system architecture.
What are some common applications of C and C++ in the tech industry?
C is often used in system programming, embedded systems, and developing operating systems. Famous operating systems like Windows, Linux, and MacOS have components written in C due to its efficiency and power. C++ is frequently utilized in software requiring object-oriented programming, such as video games, desktop applications, and real-time simulations.
Furthermore, C++ is also popular in high-performance applications like financial systems and trading platforms where speed and resource management are critical. The versatility of both languages makes them a staple in various industries, particularly those that prioritize performance and efficiency.
C , C++ PROGRAMMING LANGUAGES WITH DSA 💻 Telegram Channel
Are you looking to enhance your programming skills in C and C++ while diving into the world of Data Structures and Algorithms? Look no further than the Telegram channel 'C , C++ PROGRAMMING LANGUAGE WITH DSA 💻'! Managed by the knowledgeable and experienced user @Pradeep_saii, this channel is dedicated to providing educational content on C programming language, C++, and DSA
Whether you are a beginner looking to learn the basics of programming or an experienced coder wanting to delve deeper into data structures and algorithms, this channel has something for everyone. From tutorials and coding exercises to tips and tricks, you will find a wealth of resources to help you improve your skills
It is essential to note that all content shared on this channel is for educational purposes only, and no copyrighted material is uploaded here. So you can rest assured that you are learning in a safe and legal environment
Join the 'C , C++ PROGRAMMING LANGUAGE WITH DSA 💻' Telegram channel today and take your programming knowledge to the next level! Don't miss out on this valuable opportunity to learn and grow in the world of software development. #c_programming_language #cpp #dsa #datastructuresalgorithms #faang
C , C++ PROGRAMMING LANGUAGES WITH DSA 💻 Latest Posts
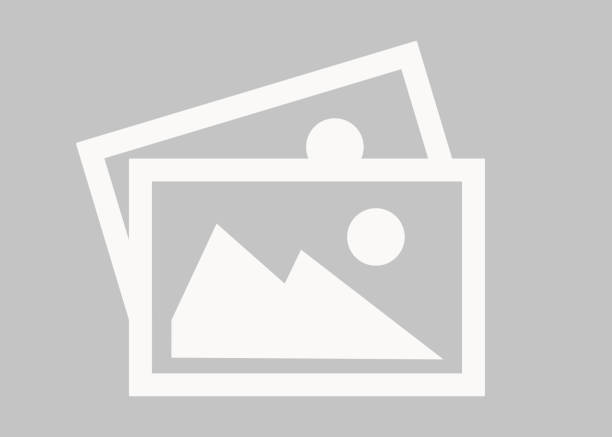
#include <iostream>
using namespace std;
int* search(int arr[][3], int rows, int cols, int target) {
static int result[2];
result[0] = -1;
result[1] = -1;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (arr[i][j] == target) {
result[0] = i;
result[1] = j;
return result;
}
}
}
return result;
}
int main() {
int arr[3][3] = {
{20, 45, 10},
{32, 26, 22},
{47, 98, 37}
};
int target;
cout << "Enter target element: ";
cin >> target;
int* result = search(arr, 3, 3, target);
if (result[0] != -1) {
cout << "Element found at index (" << result[0] << "," << result[1] << ")" << endl;
} else {
cout << "Element not found" << endl;
}
return 0;
}
#linearsearch
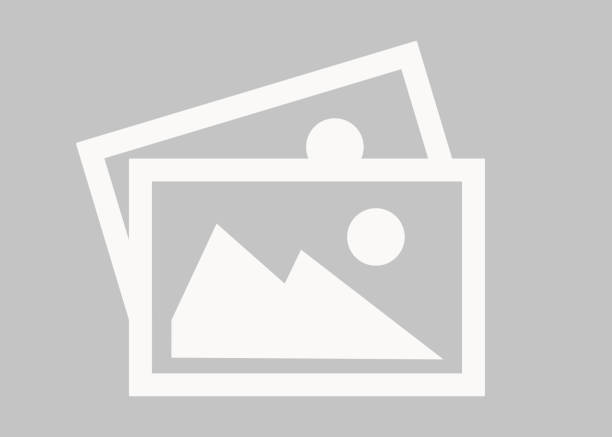
#include <iostream>
using namespace std;
int findMax(int arr[], int size) {
int max = arr[0];
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
int main() {
int arr[] = {12, 32, 23, 11, 39};
int size = sizeof(arr) / sizeof(arr[0]);
int maxNumber = findMax(arr, size);
cout << "Maximum number in array: " << maxNumber << endl;
return 0;
}
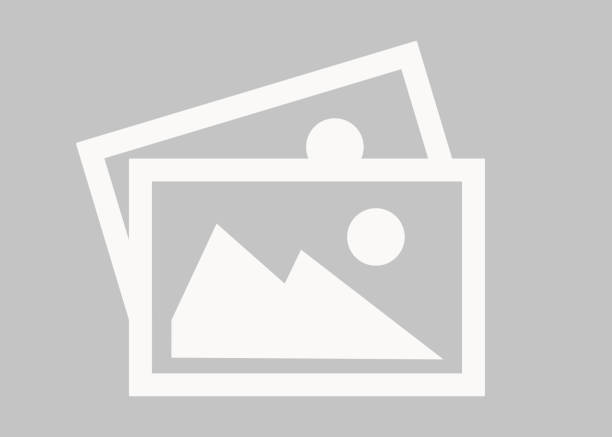
#include <iostream>
using namespace std;
int findMin(int arr[], int size) {
int min = arr[0];
for (int i = 1; i < size; i++) {
if (arr[i] < min) {
min = arr[i];
}
}
return min;
}
int main() {
int arr[] = {12, 32, 23, 11, 39};
int size = sizeof(arr) / sizeof(arr[0]);
int minNumber = findMin(arr, size);
cout << "Minimum number in array: " << minNumber << endl;
return 0;
}